Table of Contents
Real-time data processing is crucial for dynamic bidding in the ad tech industry. This article explores the key aspects of real-time data stream processing, its challenges, and solutions, providing insights for CTOs, software development managers, and product managers in ad tech startups and medium-sized companies.
Understanding Real-Time Data Stream Processing
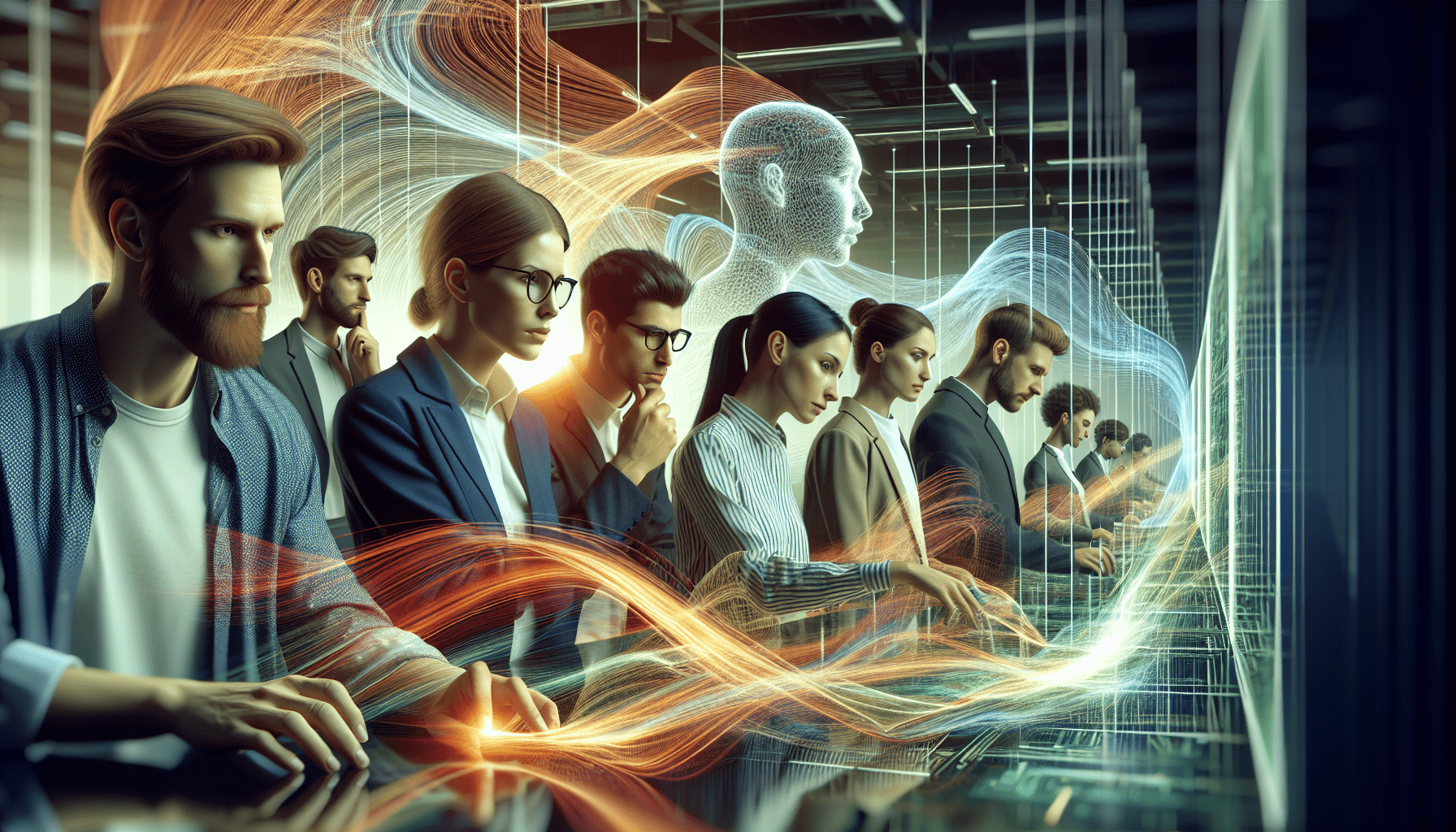
Key Points
- Real-time data processing is essential for dynamic bidding in ad tech.
- It involves handling large volumes of data with low latency.
- Challenges include data synchronization and ensuring data accuracy.
- Solutions often involve distributed systems and stream processing frameworks.
- Effective real-time processing can significantly improve bidding efficiency and accuracy.
Definition and Importance
Real-time data processing refers to the continuous input, processing, and output of data in a short time frame, typically milliseconds. This capability is vital in dynamic bidding, where decisions must be made almost instantaneously to place bids on digital advertising inventory. The speed and accuracy of these decisions can directly impact the success of advertising campaigns.
In the context of ad tech, real-time data processing enables the evaluation of bid requests, user interactions, and other relevant data points in real-time. This allows for more precise targeting and better allocation of advertising budgets, ultimately leading to higher conversion rates and return on investment (ROI).
Implementing real-time data processing requires robust infrastructure and advanced technologies. Distributed systems, stream processing frameworks, and scalable data storage solutions are commonly used to handle the high volume and velocity of data involved in dynamic bidding.
Technologies and Frameworks
Several technologies and frameworks are essential for real-time data processing in dynamic bidding. Apache Kafka is a popular choice for building real-time data pipelines due to its high throughput and fault-tolerant capabilities. Kafka Streams, a stream processing library, is often used to process data in real-time within Kafka.
Another widely used framework is Apache Flink, which provides low-latency stream processing and supports complex event processing. Flink’s ability to handle stateful computations makes it suitable for dynamic bidding scenarios where maintaining the state of user interactions is crucial.
Other technologies like Apache Spark Streaming and Google Cloud Dataflow also play significant roles in real-time data processing. These frameworks offer various features and integrations that can be tailored to specific use cases in dynamic bidding.
Challenges and Solutions
Real-time data processing for dynamic bidding presents several challenges. One of the primary challenges is ensuring data accuracy and consistency across distributed systems. Data synchronization between different data centers and handling out-of-order events are critical aspects that need to be addressed.
Another challenge is managing the high volume of data generated by bid requests and user interactions. Efficiently processing and storing this data requires scalable infrastructure and optimized data processing pipelines. Additionally, maintaining low latency is crucial to ensure timely bid responses.
Solutions to these challenges often involve using advanced data processing techniques and technologies. Implementing a multi-data center architecture with data synchronization mechanisms can help ensure data consistency. Utilizing stream processing frameworks with built-in support for stateful computations and fault tolerance can address the challenges of high data volume and low latency.
Challenges in Real-Time Data Processing for Dynamic Bidding
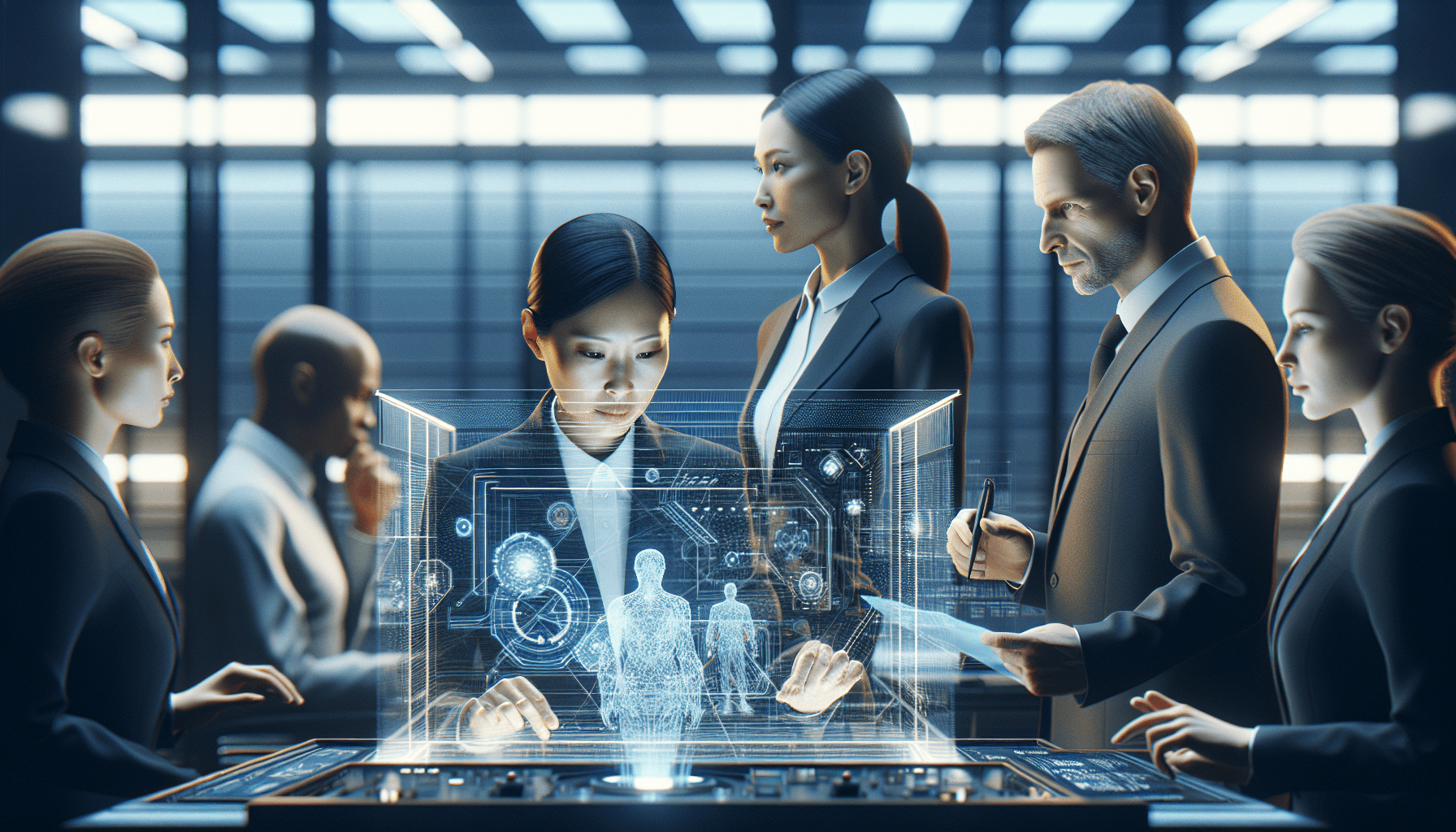
Data Synchronization Issues
Data synchronization is a significant challenge in real-time data processing for dynamic bidding. In a distributed system, data is often generated and processed across multiple data centers. Ensuring that data is synchronized and consistent across these data centers is crucial for accurate bidding decisions.
One common issue is handling out-of-order events. Bid requests and user interactions may arrive at different times and from different locations, leading to potential inconsistencies. Implementing mechanisms to reorder and synchronize these events is essential to maintain data accuracy.
Handling High Data Volume
High data volume is another challenge in real-time data processing for dynamic bidding. The ad tech industry generates vast amounts of data from bid requests, user interactions, and other sources. Processing this data in real-time requires scalable infrastructure and efficient data processing pipelines.
To handle high data volume, it is essential to use distributed systems and stream processing frameworks that can scale horizontally. Technologies like Apache Kafka and Apache Flink are designed to handle large-scale data processing and can be used to build robust real-time data pipelines.
Maintaining Low Latency
Low latency is critical in real-time data processing for dynamic bidding. Bidding decisions need to be made within milliseconds to ensure timely responses to bid requests. High latency can result in missed opportunities and reduced bidding efficiency.
To maintain low latency, it is important to optimize data processing pipelines and minimize the time taken for data ingestion, processing, and output. Using in-memory data processing techniques and optimizing network communication can help reduce latency and improve the overall performance of the bidding system.
Implementing Real-Time Data Processing for Dynamic Bidding
Step 1: Setting Up the Infrastructure
Setting up the infrastructure is the first step in implementing real-time data processing for dynamic bidding. This involves selecting the appropriate technologies and frameworks, configuring the data processing pipelines, and ensuring that the infrastructure can scale to handle high data volume.
Start by choosing a distributed messaging system like Apache Kafka to handle data ingestion and transport. Set up Kafka clusters to ensure high availability and fault tolerance. Next, select a stream processing framework like Apache Flink or Kafka Streams to process the data in real-time.
Step 2: Building Data Processing Pipelines
Building data processing pipelines is the next step. This involves defining the data flow, implementing data processing logic, and ensuring that the pipelines can handle the required data volume and latency.
Define the data flow from data ingestion to processing and output. Use stream processing frameworks to implement the data processing logic, such as filtering, aggregation, and transformation. Ensure that the pipelines are optimized for low latency and can scale horizontally to handle high data volume.
Step 3: Ensuring Data Accuracy and Consistency
Ensuring data accuracy and consistency is the final step. This involves implementing mechanisms to handle data synchronization, out-of-order events, and data consistency across distributed systems.
Use techniques like event reordering and data synchronization to ensure that data is consistent across different data centers. Implement fault-tolerant mechanisms to handle failures and ensure that data processing continues without interruptions. Regularly monitor and validate the data to ensure accuracy and consistency.
Code Example: Real-Time Data Processing with Apache Kafka and Flink
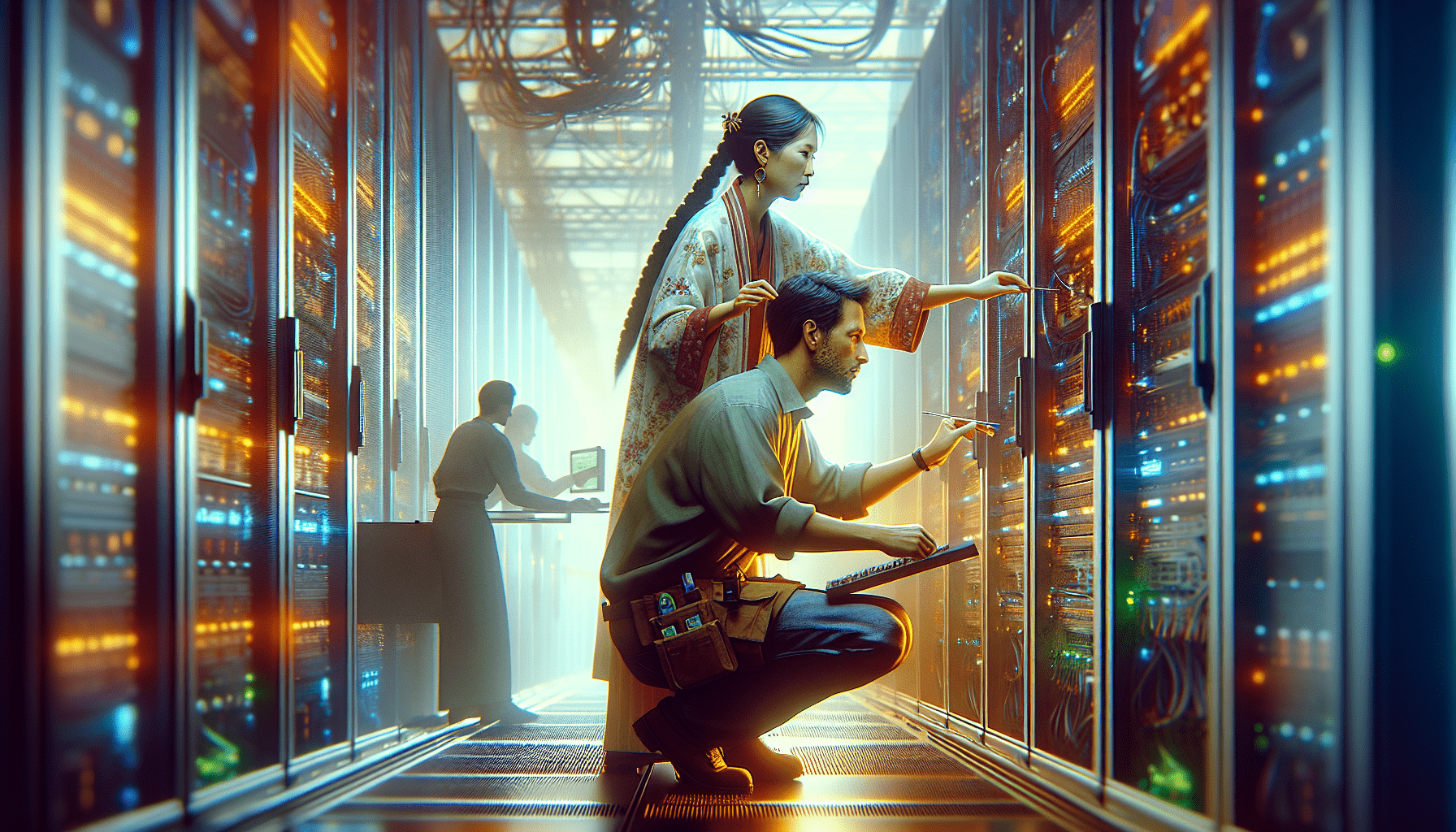
In this section, we will provide a code example demonstrating real-time data processing for dynamic bidding using Apache Kafka and Apache Flink. The example will include setting up Kafka for data ingestion and using Flink for stream processing.
import org.apache.flink.api.common.functions.MapFunction;
import org.apache.flink.streaming.api.datastream.DataStream;
import org.apache.flink.streaming.api.environment.StreamExecutionEnvironment;
import org.apache.flink.streaming.connectors.kafka.FlinkKafkaConsumer;
import org.apache.flink.streaming.connectors.kafka.FlinkKafkaProducer;
import org.apache.kafka.clients.consumer.ConsumerConfig;
import org.apache.kafka.clients.producer.ProducerConfig;
import org.apache.kafka.common.serialization.StringDeserializer;
import org.apache.kafka.common.serialization.StringSerializer;
import java.util.Properties;
public class RealTimeBiddingProcessor {
public static void main(String[] args) throws Exception {
// Set up the execution environment
final StreamExecutionEnvironment env = StreamExecutionEnvironment.getExecutionEnvironment();
// Set up Kafka consumer properties
Properties consumerProperties = new Properties();
consumerProperties.setProperty(ConsumerConfig.BOOTSTRAP_SERVERS_CONFIG, "localhost:9092");
consumerProperties.setProperty(ConsumerConfig.GROUP_ID_CONFIG, "bidding-group");
consumerProperties.setProperty(ConsumerConfig.KEY_DESERIALIZER_CLASS_CONFIG, StringDeserializer.class.getName());
consumerProperties.setProperty(ConsumerConfig.VALUE_DESERIALIZER_CLASS_CONFIG, StringDeserializer.class.getName());
// Set up Kafka producer properties
Properties producerProperties = new Properties();
producerProperties.setProperty(ProducerConfig.BOOTSTRAP_SERVERS_CONFIG, "localhost:9092");
producerProperties.setProperty(ProducerConfig.KEY_SERIALIZER_CLASS_CONFIG, StringSerializer.class.getName());
producerProperties.setProperty(ProducerConfig.VALUE_SERIALIZER_CLASS_CONFIG, StringSerializer.class.getName());
// Create Kafka consumer
FlinkKafkaConsumer kafkaConsumer = new FlinkKafkaConsumer<>("bid-requests", new SimpleStringSchema(), consumerProperties);
// Create Kafka producer
FlinkKafkaProducer kafkaProducer = new FlinkKafkaProducer<>("bid-responses", new SimpleStringSchema(), producerProperties);
// Create data stream from Kafka consumer
DataStream bidRequests = env.addSource(kafkaConsumer);
// Process bid requests
DataStream bidResponses = bidRequests.map(new MapFunction<string, string="">() {
@Override
public String map(String value) throws Exception {
// Process bid request and generate bid response
return processBidRequest(value);
}
});
// Send bid responses to Kafka producer
bidResponses.addSink(kafkaProducer);
// Execute the Flink job
env.execute("Real-Time Bidding Processor");
}
/**
* Process bid request and generate bid response.
*
* @param bidRequest the bid request
* @return the bid response
*/
private static String processBidRequest(String bidRequest) {
// Implement bid request processing logic here
// For simplicity, we return a dummy bid response
return "Bid response for: " + bidRequest;
}
}
Code language: Java (java)
This code example demonstrates how to set up a real-time data processing pipeline for dynamic bidding using Apache Kafka and Apache Flink. The Kafka consumer reads bid requests from the “bid-requests” topic, processes them using Flink, and sends the bid responses to the “bid-responses” topic using the Kafka producer.
FAQs
What is real-time data processing in dynamic bidding?
Real-time data processing in dynamic bidding involves continuously processing data from bid requests and user interactions to make instant bidding decisions. This ensures timely and accurate bids, improving the efficiency and effectiveness of advertising campaigns.
Why is low latency important in real-time data processing?
Low latency is crucial because bidding decisions need to be made within milliseconds. High latency can result in missed opportunities and reduced bidding efficiency, impacting the overall performance of the advertising platform.
What technologies are commonly used for real-time data processing?
Technologies like Apache Kafka, Apache Flink, and Apache Spark Streaming are commonly used for real-time data processing. These frameworks provide the necessary tools and capabilities to handle high data volume and low latency requirements in dynamic bidding.
How can data accuracy and consistency be ensured in real-time data processing?
Data accuracy and consistency can be ensured by implementing mechanisms for data synchronization, handling out-of-order events, and using fault-tolerant systems. Regular monitoring and validation of data also help maintain accuracy and consistency.
Future Trends in Real-Time Data Processing for Dynamic Bidding
The future of real-time data processing in dynamic bidding is promising, with several trends shaping the industry. Here are five predictions based on current trends and advancements:
- Increased use of AI and machine learning: AI and machine learning will play a significant role in optimizing bidding strategies and improving targeting accuracy.
- Greater emphasis on data privacy: With increasing regulations, there will be a stronger focus on ensuring data privacy and compliance in real-time data processing.
- Advancements in edge computing: Edge computing will enable faster data processing by bringing computation closer to the data source, reducing latency.
- Integration of blockchain technology: Blockchain can provide transparency and security in the bidding process, ensuring data integrity and trust.
- Enhanced real-time analytics: Real-time analytics will become more sophisticated, providing deeper insights and enabling more informed bidding decisions.
More Information
- Our real-time data processing – part 2 | RTB House Technical Blog: Detailed insights into the real-time data processing infrastructure at RTB House.
- Introduction to real-time bidding (RTB) – Authorized Buyers Help: An overview of real-time bidding and its importance in digital advertising.
Disclaimer
This is an AI-generated article intended for educational purposes. It does not provide advice or recommendations for implementation. The goal is to inspire readers to research and delve deeper into the topics covered.