Table of Contents
In the fast-paced world of real-time bidding (RTB), advanced feature engineering is crucial for developing predictive models that can optimize ad placements and maximize returns. This article delves into the intricacies of feature engineering in RTB, offering insights and practical steps for industry professionals.
Understanding Advanced Feature Engineering in RTB
Key Points
- Importance of feature engineering in RTB.
- Common techniques used in feature engineering.
- Challenges faced in the process.
- Solutions to overcome these challenges.
- Future trends in feature engineering for RTB.
Importance of Feature Engineering
Feature engineering is the process of using domain knowledge to create features that make machine learning algorithms work better. In RTB, this involves transforming raw data into meaningful features that can improve the accuracy of predictive models. Effective feature engineering can significantly enhance the performance of RTB systems, leading to better ad placements and higher returns on investment.
One of the primary goals of feature engineering in RTB is to identify and create features that capture the underlying patterns in the data. This can include user behavior, ad characteristics, and contextual information. By leveraging these features, predictive models can make more informed decisions about which ads to display and how much to bid.
Another critical aspect of feature engineering is the ability to handle large volumes of data in real-time. RTB systems must process vast amounts of data quickly and efficiently to make split-second decisions. Advanced feature engineering techniques, such as dimensionality reduction and feature selection, can help streamline this process and improve the overall performance of the system.
Common Techniques in Feature Engineering
Several techniques are commonly used in feature engineering for RTB. One of the most widely used methods is feature extraction, which involves transforming raw data into a set of features that can be used by machine learning algorithms. This can include techniques such as principal component analysis (PCA) and singular value decomposition (SVD).
Another common technique is feature selection, which involves identifying the most relevant features for a given predictive model. This can be done using methods such as recursive feature elimination (RFE) and mutual information. By selecting the most important features, predictive models can be more efficient and accurate.
Feature transformation is also a crucial aspect of feature engineering. This involves transforming existing features into new ones that can better capture the underlying patterns in the data. Techniques such as normalization, scaling, and encoding can be used to transform features and improve the performance of predictive models.
Challenges in Feature Engineering for RTB
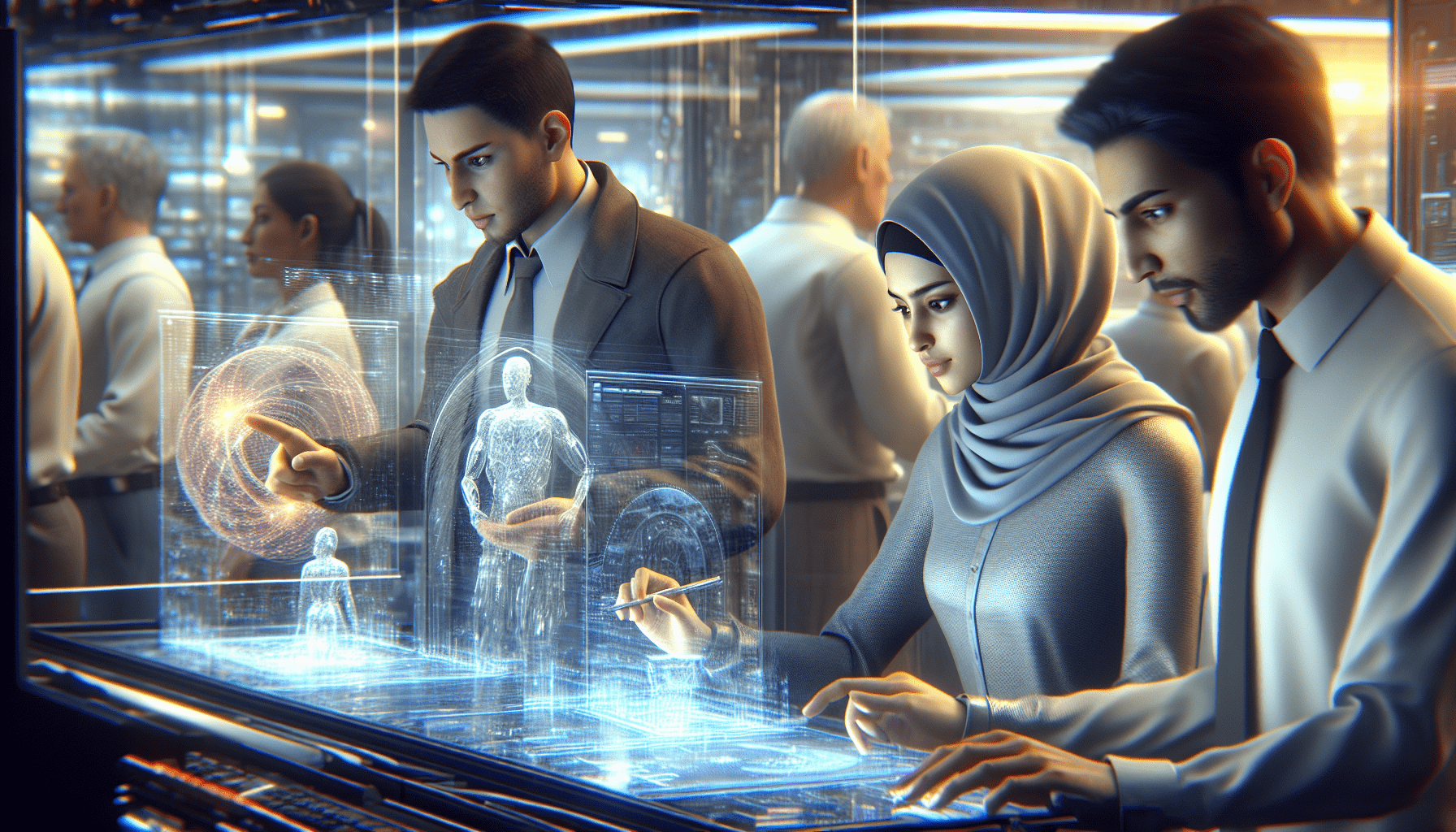
Data Quality and Consistency
One of the most significant challenges in feature engineering for RTB is ensuring data quality and consistency. RTB systems rely on vast amounts of data from various sources, and any inconsistencies or errors in the data can significantly impact the performance of predictive models. Ensuring data quality involves cleaning and preprocessing the data to remove any errors or inconsistencies.
Another aspect of data quality is dealing with missing or incomplete data. In RTB, it is common to encounter missing values or incomplete data points. Handling these issues requires advanced techniques such as imputation and data augmentation to fill in the gaps and ensure the data is complete and accurate.
Scalability and Real-Time Processing
Scalability and real-time processing are critical challenges in feature engineering for RTB. RTB systems must process vast amounts of data in real-time to make split-second decisions. This requires efficient algorithms and techniques that can handle large volumes of data quickly and accurately.
One approach to addressing this challenge is to use distributed computing frameworks such as Apache Spark and Hadoop. These frameworks can process large datasets in parallel, allowing RTB systems to scale and handle real-time data processing more efficiently. Additionally, techniques such as online learning and incremental updates can help ensure that predictive models are continuously updated with the latest data.
Feature Selection and Dimensionality Reduction
Feature selection and dimensionality reduction are essential for improving the performance of predictive models in RTB. With vast amounts of data and numerous features, it is crucial to identify the most relevant features and reduce the dimensionality of the data to improve model efficiency and accuracy.
Techniques such as recursive feature elimination (RFE) and mutual information can be used to select the most important features. Additionally, dimensionality reduction techniques such as principal component analysis (PCA) and singular value decomposition (SVD) can help reduce the number of features while retaining the most important information.
Implementing Advanced Feature Engineering in RTB
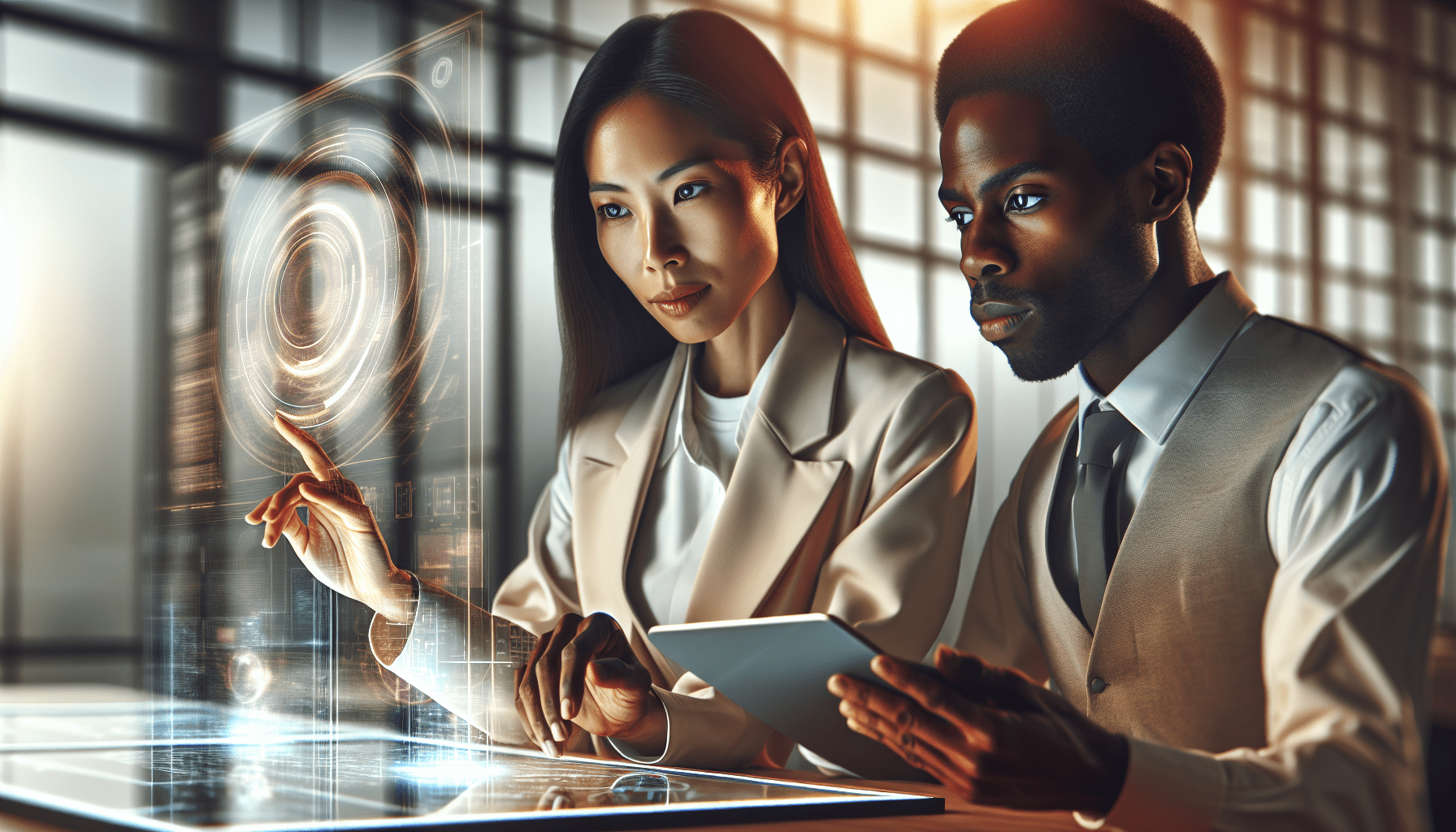
Step 1: Data Collection and Preprocessing
The first step in implementing advanced feature engineering in RTB is data collection and preprocessing. This involves gathering data from various sources, cleaning and preprocessing the data to remove any errors or inconsistencies, and handling missing or incomplete data points.
Data preprocessing techniques such as normalization, scaling, and encoding can be used to transform the data into a format suitable for feature engineering. Additionally, techniques such as imputation and data augmentation can be used to fill in any missing values and ensure the data is complete and accurate.
Step 2: Feature Extraction and Transformation
The next step is feature extraction and transformation. This involves transforming raw data into a set of features that can be used by machine learning algorithms. Techniques such as principal component analysis (PCA) and singular value decomposition (SVD) can be used to extract features from the data.
Feature transformation techniques such as normalization, scaling, and encoding can be used to transform existing features into new ones that better capture the underlying patterns in the data. This can help improve the performance of predictive models and make them more efficient and accurate.
Step 3: Feature Selection and Model Training
The final step is feature selection and model training. This involves identifying the most relevant features for a given predictive model and training the model using these features. Techniques such as recursive feature elimination (RFE) and mutual information can be used to select the most important features.
Once the features have been selected, the predictive model can be trained using machine learning algorithms such as logistic regression, decision trees, and neural networks. The model can then be evaluated and fine-tuned to ensure it performs well on the given data.
Code Example: Implementing Feature Engineering in RTB
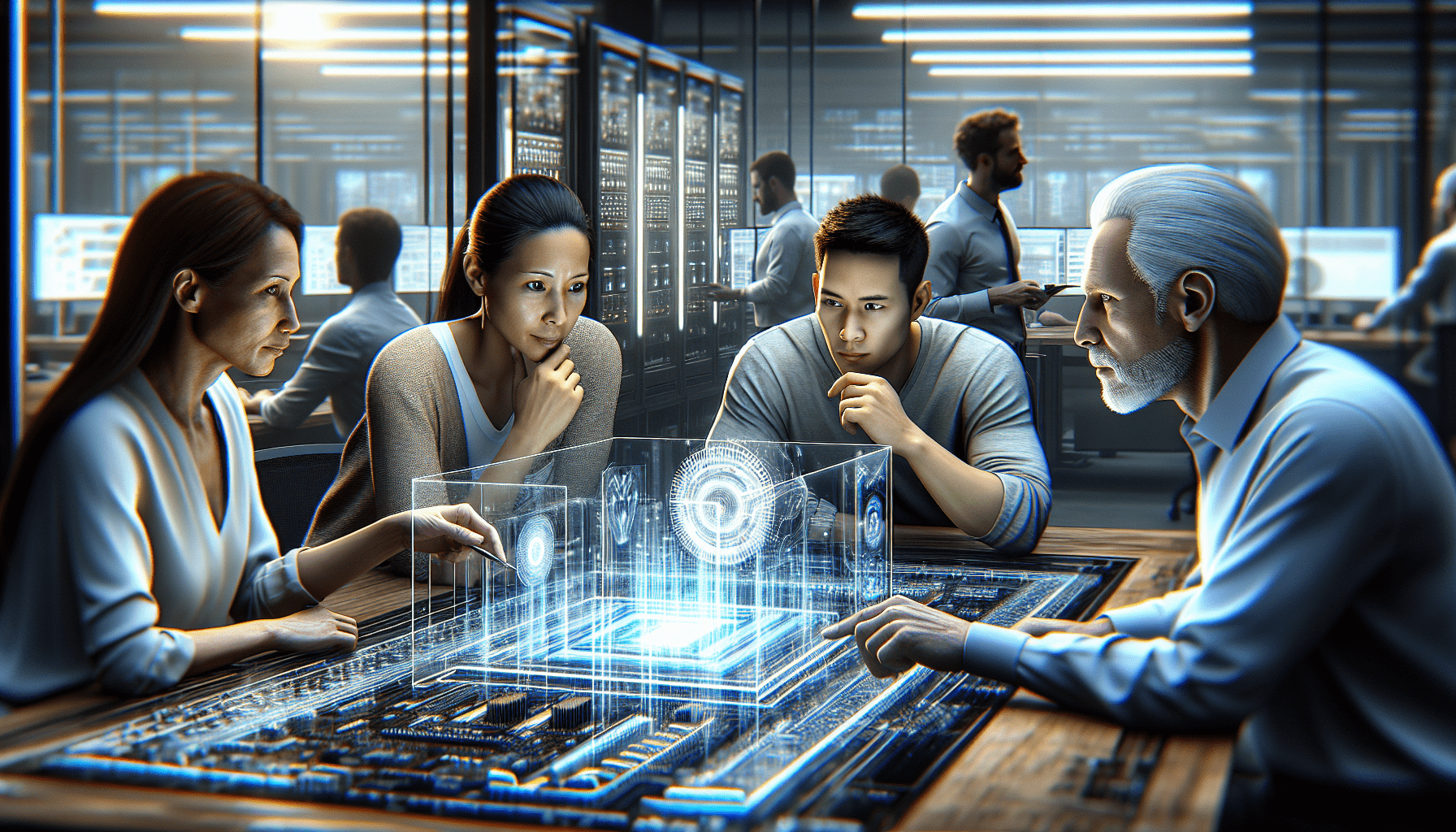
In this section, we will provide a code example that demonstrates how to implement advanced feature engineering for RTB using Python. We will use libraries such as pandas, scikit-learn, and numpy to preprocess the data, extract and transform features, and train a predictive model.
import pandas as pd
import numpy as np
from sklearn.preprocessing import StandardScaler, OneHotEncoder
from sklearn.decomposition import PCA
from sklearn.feature_selection import SelectKBest, mutual_info_classif
from sklearn.model_selection import train_test_split
from sklearn.linear_model import LogisticRegression
from sklearn.metrics import accuracy_score
class RTBFeatureEngineering:
def __init__(self, data):
self.data = data
def preprocess_data(self):
"""Preprocess the data by handling missing values and encoding categorical features."""
self.data.fillna(self.data.mean(), inplace=True)
categorical_features = self.data.select_dtypes(include=['object']).columns
encoder = OneHotEncoder(sparse=False)
encoded_features = encoder.fit_transform(self.data[categorical_features])
self.data = self.data.drop(categorical_features, axis=1)
self.data = pd.concat([self.data, pd.DataFrame(encoded_features)], axis=1)
def extract_features(self):
"""Extract features using PCA."""
scaler = StandardScaler()
scaled_data = scaler.fit_transform(self.data)
pca = PCA(n_components=10)
self.data = pca.fit_transform(scaled_data)
def select_features(self, target):
"""Select the most relevant features using mutual information."""
selector = SelectKBest(mutual_info_classif, k=5)
self.data = selector.fit_transform(self.data, target)
def train_model(self, target):
"""Train a logistic regression model using the selected features."""
X_train, X_test, y_train, y_test = train_test_split(self.data, target, test_size=0.2, random_state=42)
model = LogisticRegression()
model.fit(X_train, y_train)
predictions = model.predict(X_test)
accuracy = accuracy_score(y_test, predictions)
return accuracy
# Sample data
data = pd.DataFrame({
'feature1': [1, 2, 3, 4, 5],
'feature2': ['A', 'B', 'A', 'B', 'A'],
'feature3': [10, 20, 30, 40, 50],
'target': [0, 1, 0, 1, 0]
})
# Implementing the feature engineering process
rtb_fe = RTBFeatureEngineering(data.drop('target', axis=1))
rtb_fe.preprocess_data()
rtb_fe.extract_features()
rtb_fe.select_features(data['target'])
accuracy = rtb_fe.train_model(data['target'])
print(f'Model Accuracy: {accuracy}')
Code language: Python (python)
In this code example, we first preprocess the data by handling missing values and encoding categorical features. We then extract features using PCA and select the most relevant features using mutual information. Finally, we train a logistic regression model using the selected features and evaluate its accuracy.
FAQs
What is feature engineering in RTB?
Feature engineering in RTB involves transforming raw data into meaningful features that can improve the accuracy of predictive models. This process includes techniques such as feature extraction, feature selection, and feature transformation.
Why is feature engineering important in RTB?
Feature engineering is crucial in RTB because it helps create features that capture the underlying patterns in the data. This leads to more accurate predictive models, better ad placements, and higher returns on investment.
What are some common techniques used in feature engineering?
Common techniques in feature engineering include feature extraction (e.g., PCA, SVD), feature selection (e.g., RFE, mutual information), and feature transformation (e.g., normalization, scaling, encoding).
How can I handle missing data in RTB?
Handling missing data in RTB can be done using techniques such as imputation and data augmentation. Imputation involves filling in missing values with estimated values, while data augmentation involves generating additional data points to fill in the gaps.
Future Trends in Feature Engineering for RTB
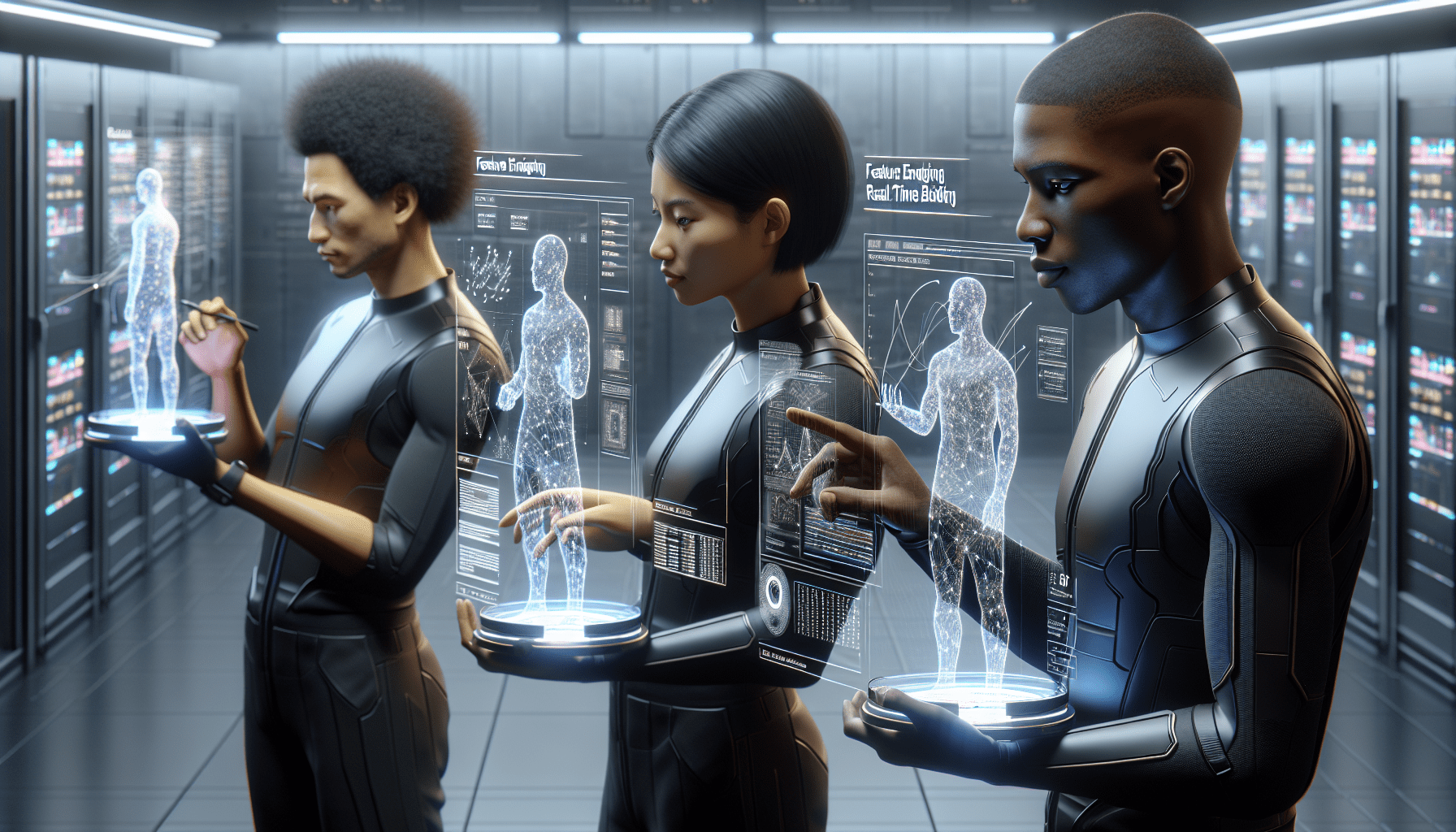
As the field of RTB continues to evolve, several trends are emerging that will shape the future of feature engineering. These trends are driven by advancements in technology, changes in user behavior, and the increasing complexity of RTB systems.
- Increased use of AI and machine learning: AI and machine learning will play a more significant role in feature engineering, enabling more sophisticated and accurate predictive models.
- Real-time feature engineering: The ability to perform feature engineering in real-time will become increasingly important, allowing RTB systems to make faster and more accurate decisions.
- Automated feature engineering: Automation tools and techniques will streamline the feature engineering process, reducing the need for manual intervention and improving efficiency.
- Integration of external data sources: Incorporating data from external sources, such as social media and IoT devices, will enhance the quality and relevance of features used in predictive models.
- Focus on privacy and security: As data privacy and security concerns grow, feature engineering techniques will need to adapt to ensure compliance with regulations and protect user data.
More Information
- GitHub – wnzhang/optimal-rtb: A benchmarking framework supporting the experiments in KDD’14 paper “Optimal Real-Time Bidding for Display Advertising” – A GitHub repository providing a benchmarking framework for RTB experiments.
- All hail the RTB Champion. Hear Ye, Hear Ye! Allow me to regale… | by Christopher Faria | QuickBooks Engineering – An article discussing the role of the RTB champion in software development teams.
- Roku – The evolution of ML in advertising: early years of RTB – An article exploring the early years of machine learning in RTB advertising.
Disclaimer
This is an AI-generated article with educative purposes and doesn’t intend to give advice or recommend its implementation. The goal is to inspire readers to research and delve deeper into the topics covered in the article.
- From SaaS to AI Agents - 05/27/25
- The AI Automation Engineer - 05/13/25
- Hire One Developer to Press One Key - 05/06/25