Table of Contents
Graph Neural Networks (GNNs) are revolutionizing Real-Time Bidding (RTB) systems in the ad tech industry.
This article explores how GNNs can enhance RTB systems, providing a robust solution for developing AI-powered advertising platforms.
Understanding Graph Neural Networks for RTB Systems
Key Points
- Graph Neural Networks (GNNs) can significantly improve the performance of RTB systems.
- GNNs help in detecting fraudulent activities in real-time.
- They enable better user targeting by analyzing complex relationships.
- GNNs can process large-scale data efficiently.
- Implementing GNNs requires a deep understanding of both graph theory and neural networks.
What are Graph Neural Networks?
Graph Neural Networks (GNNs) are a type of neural network designed to work with graph-structured data. Unlike traditional neural networks, which operate on fixed-size input data, GNNs can process data represented as graphs, making them ideal for applications where relationships between data points are crucial.
GNNs use nodes to represent entities and edges to represent relationships between these entities. This structure allows GNNs to capture complex dependencies and interactions within the data, making them highly effective for tasks like social network analysis, recommendation systems, and fraud detection.
In the context of RTB systems, GNNs can analyze the intricate relationships between users, advertisers, and content, enabling more accurate and efficient bidding strategies.
How GNNs Work
GNNs operate by iteratively updating the representation of each node based on its neighbors. This process, known as message passing, allows the network to aggregate information from the entire graph, leading to a comprehensive understanding of the data.
Each iteration involves two main steps: aggregation and update. During aggregation, a node collects information from its neighbors. In the update step, the node’s representation is updated based on the aggregated information. This process is repeated for a fixed number of iterations, allowing the network to capture long-range dependencies within the graph.
GNNs can be trained using supervised, unsupervised, or semi-supervised learning methods, depending on the availability of labeled data. The choice of training method and the design of the network architecture are critical factors that influence the performance of GNNs.
Applications of GNNs in RTB Systems
GNNs have several applications in RTB systems, including fraud detection, user targeting, and bid optimization. By analyzing the relationships between users, advertisers, and content, GNNs can identify patterns and anomalies that traditional methods might miss.
For instance, GNNs can detect fraudulent activities by identifying unusual patterns in the bidding behavior of certain users or advertisers. This capability is crucial for maintaining the integrity of RTB systems and ensuring that advertisers get the best value for their money.
Additionally, GNNs can enhance user targeting by analyzing the complex relationships between users and content. This analysis enables RTB systems to deliver more relevant ads to users, improving the overall effectiveness of advertising campaigns.
Challenges in the Ad Tech Industry
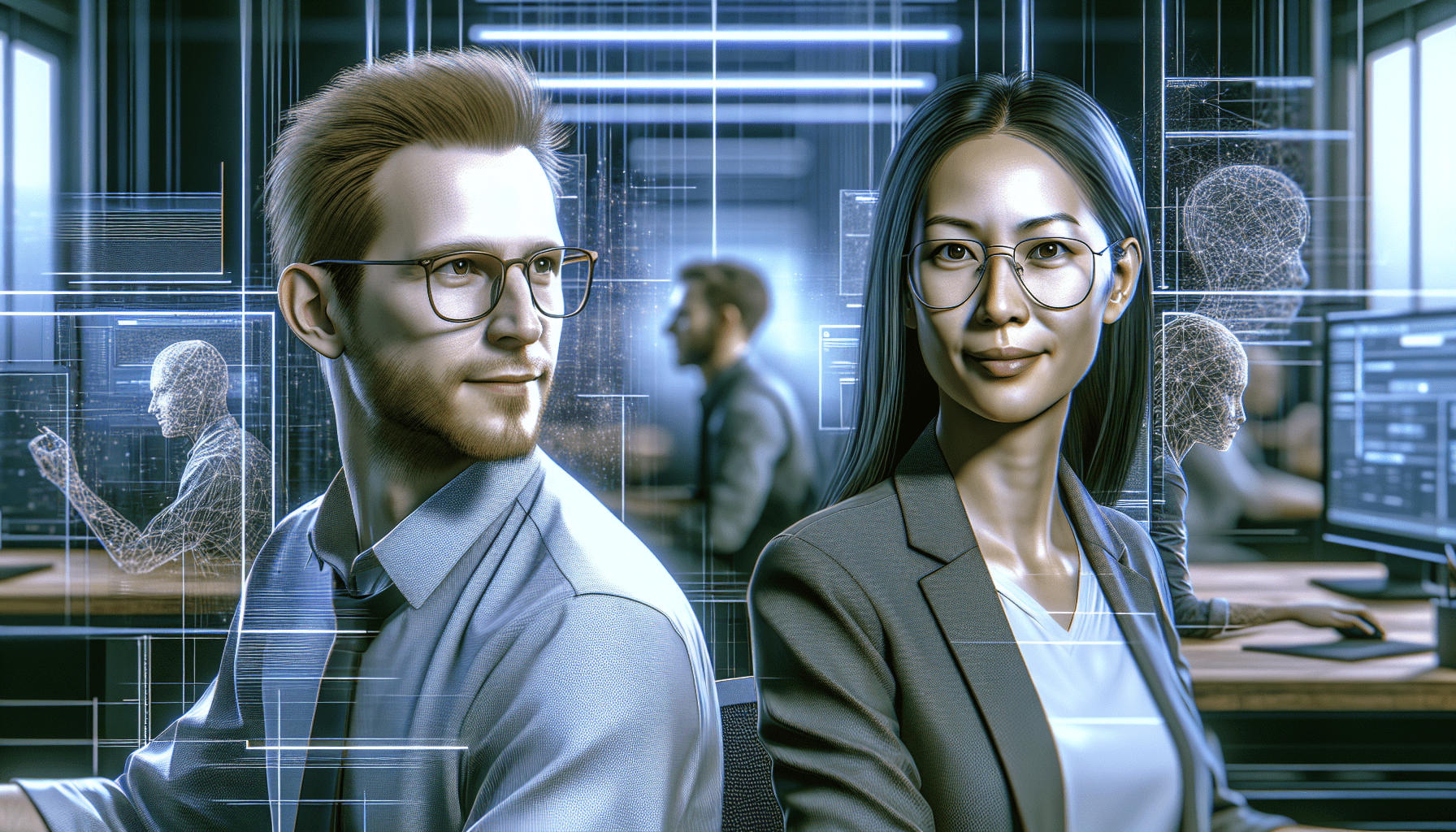
Fraud Detection
Fraud detection is one of the most significant challenges in the ad tech industry. Fraudulent activities, such as click fraud and impression fraud, can lead to substantial financial losses for advertisers and undermine the credibility of RTB systems.
Traditional fraud detection methods often struggle to keep up with the evolving tactics of fraudsters. These methods typically rely on predefined rules and patterns, which can be easily circumvented by sophisticated fraud schemes. As a result, there is a growing need for more advanced and adaptive fraud detection techniques.
Data Scalability
RTB systems must process vast amounts of data in real-time to make bidding decisions. This requirement poses a significant challenge in terms of data scalability. The ability to efficiently process and analyze large-scale data is crucial for the success of RTB systems.
Traditional data processing methods often fall short in handling the sheer volume and complexity of data involved in RTB systems. This limitation can lead to delays in bidding decisions and reduced effectiveness of advertising campaigns. Therefore, there is a need for more scalable and efficient data processing techniques.
User Privacy
Maintaining user privacy is a critical concern in the ad tech industry. RTB systems rely on user data to deliver targeted ads, but the collection and use of this data must comply with privacy regulations and user expectations.
Balancing the need for personalized advertising with the requirement to protect user privacy is a complex challenge. RTB systems must implement robust privacy measures to ensure that user data is handled responsibly and transparently. Failure to do so can result in legal repercussions and damage to the reputation of the ad tech company.
Implementing GNNs to Solve Ad Tech Challenges
Step 1: Data Preparation
The first step in implementing GNNs for RTB systems is data preparation. This step involves collecting and preprocessing the data to create a graph representation that can be used by the GNN.
Data preparation includes tasks such as data cleaning, feature extraction, and graph construction. The quality of the data and the accuracy of the graph representation are critical factors that influence the performance of the GNN. Therefore, it is essential to invest time and effort in this step to ensure that the data is well-prepared.
Step 2: Model Design
The next step is to design the GNN model. This step involves selecting the appropriate architecture and hyperparameters for the GNN. The choice of architecture depends on the specific requirements of the RTB system and the nature of the data.
Common GNN architectures include Graph Convolutional Networks (GCNs), Graph Attention Networks (GATs), and GraphSAGE. Each architecture has its strengths and weaknesses, and the choice of architecture should be based on a thorough understanding of the problem and the data. Additionally, hyperparameters such as the number of layers, learning rate, and batch size must be carefully tuned to achieve optimal performance.
Step 3: Training and Evaluation
The final step is to train and evaluate the GNN model. This step involves using the prepared data to train the GNN and evaluate its performance on a validation set.
Training the GNN involves optimizing the model parameters using a suitable loss function and optimization algorithm. The performance of the GNN is evaluated using metrics such as accuracy, precision, recall, and F1-score. It is essential to monitor these metrics during training to ensure that the model is learning effectively and to make any necessary adjustments to the training process.
Code Example: Implementing GNN for Fraud Detection in RTB Systems
In this section, we will provide a code example of implementing a GNN for fraud detection in RTB systems using Python and the PyTorch Geometric library.
import torch
import torch.nn.functional as F
from torch_geometric.nn import GCNConv
from torch_geometric.data import Data
class FraudDetectionGNN(torch.nn.Module):
def __init__(self, num_node_features, num_classes):
super(FraudDetectionGNN, self).__init__()
self.conv1 = GCNConv(num_node_features, 16)
self.conv2 = GCNConv(16, num_classes)
def forward(self, data):
x, edge_index = data.x, data.edge_index
x = self.conv1(x, edge_index)
x = F.relu(x)
x = F.dropout(x, training=self.training)
x = self.conv2(x, edge_index)
return F.log_softmax(x, dim=1)
def load_data():
# Sample data
edge_index = torch.tensor([[0, 1, 1, 2], [1, 0, 2, 1]], dtype=torch.long)
x = torch.tensor([[1, 0], [0, 1], [1, 1]], dtype=torch.float)
y = torch.tensor([0, 1, 0], dtype=torch.long)
data = Data(x=x, edge_index=edge_index, y=y)
return data
def train(model, data, epochs=100):
optimizer = torch.optim.Adam(model.parameters(), lr=0.01)
model.train()
for epoch in range(epochs):
optimizer.zero_grad()
out = model(data)
loss = F.nll_loss(out[data.train_mask], data.y[data.train_mask])
loss.backward()
optimizer.step()
return model
def evaluate(model, data):
model.eval()
_, pred = model(data).max(dim=1)
correct = int(pred[data.test_mask].eq(data.y[data.test_mask]).sum().item())
acc = correct / int(data.test_mask.sum())
return acc
# Load data
data = load_data()
data.train_mask = torch.tensor([True, True, False], dtype=torch.bool)
data.test_mask = torch.tensor([False, False, True], dtype=torch.bool)
# Initialize and train model
model = FraudDetectionGNN(num_node_features=2, num_classes=2)
model = train(model, data)
# Evaluate model
accuracy = evaluate(model, data)
print(f'Accuracy: {accuracy:.4f}')
Code language: Python (python)
This code example demonstrates how to implement a GNN for fraud detection in RTB systems. The `FraudDetectionGNN` class defines the GNN architecture using two graph convolutional layers. The `load_data` function creates a sample graph dataset, and the `train` function trains the GNN model. Finally, the `evaluate` function evaluates the model’s performance on a test set.
FAQs
What are Graph Neural Networks?
Graph Neural Networks (GNNs) are a type of neural network designed to work with graph-structured data. They use nodes to represent entities and edges to represent relationships, allowing them to capture complex dependencies within the data.
How do GNNs improve RTB systems?
GNNs improve RTB systems by analyzing the relationships between users, advertisers, and content. This analysis enables more accurate bidding strategies, better user targeting, and effective fraud detection.
What are the challenges in implementing GNNs?
Challenges in implementing GNNs include data preparation, model design, and training. Ensuring the quality of data, selecting the appropriate architecture, and tuning hyperparameters are critical factors for the success of GNNs.
Which libraries are used for implementing GNNs?
Popular libraries for implementing GNNs include PyTorch Geometric, DGL (Deep Graph Library), and TensorFlow GNN. These libraries provide tools and functions to build and train GNN models efficiently.
Future of GNNs in RTB Systems
The future of GNNs in RTB systems looks promising, with several trends indicating their growing importance. Here are five predictions for the future:
- Increased Adoption: More ad tech companies will adopt GNNs to enhance their RTB systems, driven by the need for better performance and fraud detection.
- Improved Algorithms: Advances in GNN algorithms will lead to more efficient and accurate models, further boosting their effectiveness in RTB systems.
- Integration with Other AI Technologies: GNNs will be integrated with other AI technologies, such as reinforcement learning and natural language processing, to create more sophisticated RTB systems.
- Enhanced Privacy Measures: As privacy concerns grow, GNNs will incorporate advanced privacy-preserving techniques to ensure user data is handled responsibly.
- Real-Time Processing: GNNs will become more capable of real-time processing, enabling RTB systems to make faster and more accurate bidding decisions.
More Information
- Alleviating the Inconsistency Problem of Applying Graph Neural Network to Fraud Detection | Proceedings of the 43rd International ACM SIGIR Conference on Research and Development in Information Retrieval – This paper discusses the challenges and solutions for applying GNNs to fraud detection.
- Measuring and Improving the Use of Graph Information in Graph Neural Networks | OpenReview – This paper introduces metrics to measure the use of graph information in GNNs and proposes an improved GNN model.
- Enhancing Graph Neural Network-based Fraud Detectors against Camouflaged Fraudsters | Proceedings of the 29th ACM International Conference on Information & Knowledge Management – This paper explores techniques to enhance GNN-based fraud detectors against sophisticated fraudsters.
Disclaimer
This is an AI-generated article intended for educational purposes. It does not provide advice or recommendations for implementation. The goal is to inspire readers to research and delve deeper into the topics covered.
- Graph Neural Networks for RTB Systems - 10/02/24
- Measuring Emotional Impact in Local Campaigns - 09/25/24
- The Role of Mirror Neurons in Consumer Behavior - 09/18/24